How to easily create skid marks in Unity
Add trails to the rear tires of your car and create good looking skid marks with little to no effort.
Updated: Aug 4, 2023
Published: Jul 29, 2023
Easy as adding two trails
In Unity, add a trail, which is built into Unity already, to the rear tires of your car. Create a game object for your car, and then add two trails as children. Position them on the rear tires. I didn't change a lot of the default settings to create the skid marks in the main image. I adjusted the graph slightly, turned "Cast Shadows" off, and unchecked "Receive Shadows" under the Lighting tab. Finally, I changed the color to Black (see below image.)
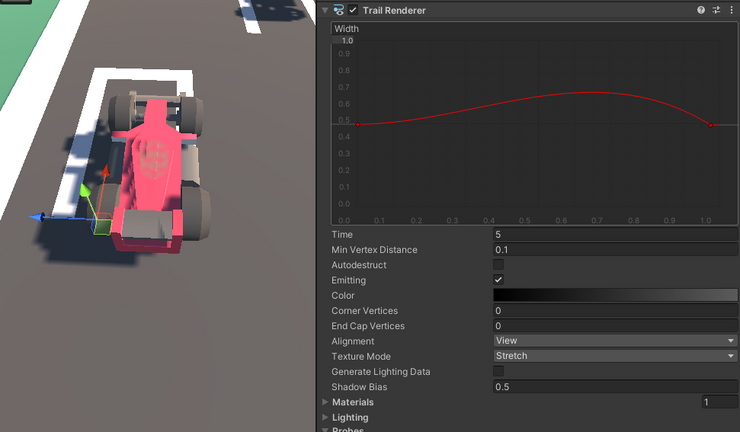
With these changes, you already have what you need to create skid marks. However, we are creating skid marks all the time. We don't want that. We only want to create them when we are turning.
In order to make it work, we need to write a little bit of code, but not too much. Create a SkidMarks.cs
file, and add the following
SkidMarks.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SkidMarks : MonoBehaviour
{
[SerializeField] Rigidbody car; // the parent rigid body
TrailRenderer trail;
private Quaternion lastRotation;
void Start()
{
trail = gameObject.GetComponent<TrailRenderer>();
trail.emitting = false;
lastRotation = car.transform.rotation;
}
void Update()
{
bool isSteering = false;
if (car.transform.hasChanged)
{
if (car.transform.rotation != lastRotation)
{
isSteering = true;
lastRotation = car.transform.rotation;
}
}
// Only emit the skid marks if we are steering
trail.emitting = isSteering;
}
}
Add the script to each trail in the car, and remember to drag the car's rigidbody into the car
slot of the script.
The script will store the rotation of the car for each update cycle, and also compare the last rotation with the new rotation to see if the car has turned or not. If the car is turning, we add skid marks by setting `trail.emitter = true`.
But, why aren't you just checking the steering input, you may be asking? And that's a valid question. The reason is because I'm creating a multiplayer game. I want to make sure all cars in the game render skid marks, not only the one we are controlling. With this technique, we don't have to sync the skid marks over the network. We just render them on the clients. It's not important if the skid marks are in sync or not.
For more granular control, I'd probably check the input instead for a single player game. We can more easily set a threshold, and only create the skid marks when we are doing sharp turns.
If you liked this blog post, or want me to elaborate on other topics, consider following me on Twitter, @ReitGames, and mention me in a Tweet. Happy coding!